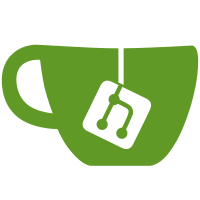
11 changed files with 36 additions and 73 deletions
@ -1,20 +0,0 @@ |
|||
// Process < > " (& was processed in markdown escape)
|
|||
|
|||
module.exports = function escape_html_char(state, silent) { |
|||
var ch = state.src.charCodeAt(state.pos), |
|||
str; |
|||
|
|||
if (ch === 0x3C/* < */) { |
|||
str = '<'; |
|||
} else if (ch === 0x3E/* > */) { |
|||
str = '>'; |
|||
} else if (ch === 0x22/* " */) { |
|||
str = '"'; |
|||
} else { |
|||
return false; |
|||
} |
|||
|
|||
if (!silent) { state.pending += str; } |
|||
state.pos++; |
|||
return true; |
|||
}; |
Loading…
Reference in new issue