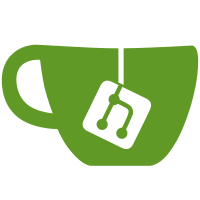
7 changed files with 228 additions and 3 deletions
@ -0,0 +1,43 @@ |
|||||
|
// Remarkable default options
|
||||
|
|
||||
|
'use strict'; |
||||
|
|
||||
|
|
||||
|
module.exports = { |
||||
|
options: { |
||||
|
html: false, // Enable html tags in source
|
||||
|
xhtmlOut: false, // Use '/' to close single tags (<br />)
|
||||
|
breaks: false, // Convert '\n' in paragraphs into <br>
|
||||
|
langPrefix: 'language-', // CSS language prefix for fenced blocks
|
||||
|
linkify: false, // autoconvert url-like texts to links
|
||||
|
typographer: false, // Enable smartypants and other sweet transforms
|
||||
|
|
||||
|
// Highlighter function. Should return escaped html,
|
||||
|
// or '' if input not changed
|
||||
|
highlight: function (/*str, , lang*/) { return ''; }, |
||||
|
|
||||
|
maxNesting: 20 // Internal protection, recursion limit
|
||||
|
}, |
||||
|
|
||||
|
components: { |
||||
|
|
||||
|
// Don't restrict block/inline rules
|
||||
|
block: {}, |
||||
|
inline: {}, |
||||
|
|
||||
|
typographer: { |
||||
|
options: { |
||||
|
singleQuotes: '‘’', // set empty to disable
|
||||
|
doubleQuotes: '“”', // set '«»' for russian, '„“' for deutch, empty to disable
|
||||
|
copyright: true, // (c) (C) → ©
|
||||
|
trademark: true, // (tm) (TM) → ™
|
||||
|
registered: true, // (r) (R) → ®
|
||||
|
plusminus: true, // +- → ±
|
||||
|
paragraph: true, // (p) (P) → §
|
||||
|
ellipsis: true, // ... → …
|
||||
|
dupes: true, // ???????? → ???, !!!!! → !!!, `,,` → `,`
|
||||
|
dashes: true // -- → —
|
||||
|
} |
||||
|
} |
||||
|
} |
||||
|
}; |
@ -0,0 +1,78 @@ |
|||||
|
// Process ++inserted text++
|
||||
|
|
||||
|
'use strict'; |
||||
|
|
||||
|
module.exports = function ins(state, silent) { |
||||
|
var found, |
||||
|
pos, |
||||
|
max = state.posMax, |
||||
|
start = state.pos, |
||||
|
lastChar, |
||||
|
nextChar; |
||||
|
|
||||
|
if (state.src.charCodeAt(start) !== 0x2B/* + */) { return false; } |
||||
|
if (start + 4 >= max) { return false; } |
||||
|
if (state.src.charCodeAt(start + 1) !== 0x2B/* + */) { return false; } |
||||
|
|
||||
|
// make ins lower a priority tag with respect to links, same as <em>;
|
||||
|
// this code also prevents recursion
|
||||
|
if (silent && state.isInLabel) { return false; } |
||||
|
|
||||
|
if (state.level >= state.options.maxNesting) { return false; } |
||||
|
|
||||
|
lastChar = start > 0 ? state.src.charCodeAt(start - 1) : -1; |
||||
|
nextChar = state.src.charCodeAt(start + 2); |
||||
|
|
||||
|
if (lastChar === 0x2B/* + */) { return false; } |
||||
|
if (nextChar === 0x2B/* + */) { return false; } |
||||
|
if (nextChar === 0x20 || nextChar === 0x0A) { return false; } |
||||
|
|
||||
|
pos = start + 2; |
||||
|
while (pos < max && state.src.charCodeAt(pos) === 0x2B/* + */) { pos++; } |
||||
|
if (pos !== start + 2) { |
||||
|
// sequence of 3+ markers taking as literal, same as in a emphasis
|
||||
|
state.pos += pos - start; |
||||
|
if (!silent) { state.pending += state.src.slice(start, pos); } |
||||
|
return true; |
||||
|
} |
||||
|
|
||||
|
state.pos = start + 2; |
||||
|
|
||||
|
while (state.pos + 1 < max) { |
||||
|
if (state.src.charCodeAt(state.pos) === 0x2B/* + */) { |
||||
|
if (state.src.charCodeAt(state.pos + 1) === 0x2B/* + */) { |
||||
|
lastChar = state.src.charCodeAt(state.pos - 1); |
||||
|
nextChar = state.pos + 2 < max ? state.src.charCodeAt(state.pos + 2) : -1; |
||||
|
if (nextChar !== 0x2B/* + */ && lastChar !== 0x2B/* + */) { |
||||
|
if (lastChar !== 0x20 && lastChar !== 0x0A) { |
||||
|
// closing '++'
|
||||
|
found = true; |
||||
|
break; |
||||
|
} |
||||
|
} |
||||
|
} |
||||
|
} |
||||
|
|
||||
|
state.parser.skipToken(state); |
||||
|
} |
||||
|
|
||||
|
if (!found) { |
||||
|
// parser failed to find ending tag, so it's not valid emphasis
|
||||
|
state.pos = start; |
||||
|
return false; |
||||
|
} |
||||
|
|
||||
|
// found!
|
||||
|
state.posMax = state.pos; |
||||
|
state.pos = start + 2; |
||||
|
|
||||
|
if (!silent) { |
||||
|
state.push({ type: 'ins_open', level: state.level++ }); |
||||
|
state.parser.tokenize(state); |
||||
|
state.push({ type: 'ins_close', level: --state.level }); |
||||
|
} |
||||
|
|
||||
|
state.pos = state.posMax + 2; |
||||
|
state.posMax = max; |
||||
|
return true; |
||||
|
}; |
@ -0,0 +1,93 @@ |
|||||
|
. |
||||
|
++Insert++ |
||||
|
. |
||||
|
<p><ins>Insert</ins></p> |
||||
|
. |
||||
|
|
||||
|
|
||||
|
These are not inserts, you have to use exactly two "++": |
||||
|
. |
||||
|
x +++foo+++ |
||||
|
|
||||
|
x ++foo+++ |
||||
|
|
||||
|
x +++foo++ |
||||
|
. |
||||
|
<p>x +++foo+++</p> |
||||
|
<p>x ++foo+++</p> |
||||
|
<p>x +++foo++</p> |
||||
|
. |
||||
|
|
||||
|
Inserts have the same priority as emphases: |
||||
|
|
||||
|
. |
||||
|
**++test**++ |
||||
|
|
||||
|
++**test++** |
||||
|
. |
||||
|
<p>**<ins>test**</ins></p> |
||||
|
<p>++<strong>test++</strong></p> |
||||
|
. |
||||
|
|
||||
|
Inserts have the same priority as emphases with respect to links: |
||||
|
. |
||||
|
[++link]()++ |
||||
|
|
||||
|
++[link++]() |
||||
|
. |
||||
|
<p><a href="">++link</a>++</p> |
||||
|
<p>++<a href="">link++</a></p> |
||||
|
. |
||||
|
|
||||
|
Inserts have the same priority as emphases with respect to backticks: |
||||
|
. |
||||
|
++`code++` |
||||
|
|
||||
|
`++code`++ |
||||
|
. |
||||
|
<p>++<code>code++</code></p> |
||||
|
<p><code>++code</code>++</p> |
||||
|
. |
||||
|
|
||||
|
Nested inserts: |
||||
|
. |
||||
|
++foo ++bar++ baz++ |
||||
|
. |
||||
|
<p><ins>foo <ins>bar</ins> baz</ins></p> |
||||
|
. |
||||
|
|
||||
|
. |
||||
|
++f **o ++o b++ a** r++ |
||||
|
. |
||||
|
<p><ins>f <strong>o <ins>o b</ins> a</strong> r</ins></p> |
||||
|
. |
||||
|
|
||||
|
Should not have a whitespace between text and "++": |
||||
|
. |
||||
|
foo ++ bar ++ baz |
||||
|
. |
||||
|
<p>foo ++ bar ++ baz</p> |
||||
|
. |
||||
|
|
||||
|
|
||||
|
Newline should be considered a whitespace: |
||||
|
|
||||
|
. |
||||
|
++test |
||||
|
++ |
||||
|
|
||||
|
++ |
||||
|
test++ |
||||
|
|
||||
|
++ |
||||
|
test |
||||
|
++ |
||||
|
. |
||||
|
<p>++test |
||||
|
++</p> |
||||
|
<p>++ |
||||
|
test++</p> |
||||
|
<p>++ |
||||
|
test |
||||
|
++</p> |
||||
|
. |
Loading…
Reference in new issue