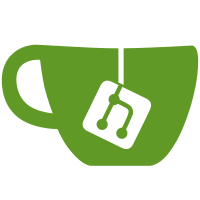
10 changed files with 171 additions and 94 deletions
@ -0,0 +1,60 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Windows; |
|||
using System.Windows.Controls; |
|||
using System.Windows.Media.Animation; |
|||
using W10SS_GUI.Controls; |
|||
using W10SS_GUI.Properties; |
|||
|
|||
namespace W10SS_GUI.Classes |
|||
{ |
|||
internal class Gui |
|||
{ |
|||
private Dictionary<string, StackPanel> _togglesCategoryPanels = new Dictionary<string, StackPanel>(); |
|||
private MainWindow MainWindow = Application.Current.MainWindow as MainWindow; |
|||
private HamburgerCategoryButton _lastclickedbutton; |
|||
|
|||
internal string LastClickedButtonName |
|||
{ |
|||
get |
|||
{ |
|||
return _lastclickedbutton.Name as string; |
|||
} |
|||
} |
|||
|
|||
internal Gui() |
|||
{ |
|||
InitializeVariables(); |
|||
} |
|||
|
|||
internal void InitializeVariables() |
|||
{ |
|||
foreach (var tagValue in Application.Current.Resources.MergedDictionaries.Where(r => r.Source.Segments[2] == "tags.xaml").FirstOrDefault().Values) |
|||
{ |
|||
_togglesCategoryPanels.Add(tagValue.ToString(), MainWindow.panelTogglesCategoryContainer.Children.OfType<StackPanel>().Where(p => p.Tag == tagValue).FirstOrDefault()); |
|||
} |
|||
} |
|||
|
|||
internal void SetActivePanel(HamburgerCategoryButton button) |
|||
{ |
|||
_lastclickedbutton = button; |
|||
|
|||
foreach (KeyValuePair<string, StackPanel> kvp in _togglesCategoryPanels) |
|||
{ |
|||
kvp.Value.Visibility = kvp.Key == button.Tag as string ? Visibility.Visible : Visibility.Collapsed; |
|||
} |
|||
|
|||
MainWindow.textTogglesHeader.Text = MainWindow.Resources[button.Name] as string; |
|||
} |
|||
|
|||
internal void SetHamburgerWidth(string cultureName) |
|||
{ |
|||
Storyboard storyboard = MainWindow.TryFindResource("animationHamburgerOpen") as Storyboard; |
|||
DoubleAnimation animation = storyboard.Children[0] as DoubleAnimation; |
|||
animation.To = cultureName == "ru" |
|||
? Convert.ToDouble(MainWindow.TryFindResource("panelHamburgerRuMaxWidth")) |
|||
: Convert.ToDouble(MainWindow.TryFindResource("panelHamburgerEnMaxWidth")); |
|||
} |
|||
} |
|||
} |
@ -0,0 +1,23 @@ |
|||
using System; |
|||
using System.Collections.Generic; |
|||
using System.Linq; |
|||
using System.Text; |
|||
using System.Threading.Tasks; |
|||
using System.Windows; |
|||
|
|||
namespace W10SS_GUI |
|||
{ |
|||
internal static class Tags |
|||
{ |
|||
internal static readonly string Privacy = string.Format("{0}", Application.Current.Resources["tagCategoryPrivacy"]); |
|||
internal static readonly string Ui = string.Format("{0}", Application.Current.Resources["tagCategoryUi"]); |
|||
internal static readonly string OneDrive = string.Format("{0}", Application.Current.Resources["tagCategoryOneDrive"]); |
|||
internal static readonly string System = string.Format("{0}", Application.Current.Resources["tagCategorySystem"]); |
|||
internal static readonly string StartMenu = string.Format("{0}", Application.Current.Resources["tagCategoryStartMenu"]); |
|||
internal static readonly string Uwp = string.Format("{0}", Application.Current.Resources["tagCategoryUwp"]); |
|||
internal static readonly string WinGame = string.Format("{0}", Application.Current.Resources["tagCategoryWinGame"]); |
|||
internal static readonly string TaskScheduler = string.Format("{0}", Application.Current.Resources["tagCategoryTaskScheduler"]); |
|||
internal static readonly string Defender = string.Format("{0}", Application.Current.Resources["tagCategoryDefender"]); |
|||
internal static readonly string ContextMenu = string.Format("{0}", Application.Current.Resources["tagCategoryContextMenu"]); |
|||
} |
|||
} |
Loading…
Reference in new issue