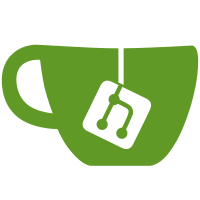
13 changed files with 289 additions and 33 deletions
@ -0,0 +1,82 @@ |
|||||
|
<UserControl x:Class="W10SS_GUI.Controls.HamburgerCategoryFlashButton" |
||||
|
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" |
||||
|
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" |
||||
|
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" |
||||
|
xmlns:d="http://schemas.microsoft.com/expression/blend/2008" |
||||
|
xmlns:local="clr-namespace:W10SS_GUI.Controls" |
||||
|
mc:Ignorable="d" |
||||
|
d:DesignHeight="{StaticResource buttonHamburgerHeight}" d:DesignWidth="{StaticResource panelHamburgerMaxWidth}"> |
||||
|
<UserControl.Resources> |
||||
|
<Style TargetType="TextBlock"> |
||||
|
<Style.Triggers> |
||||
|
<Trigger Property="IsEnabled" Value="True"> |
||||
|
<Setter Property="Foreground" Value="{DynamicResource textHamburgerForeground}" /> |
||||
|
</Trigger> |
||||
|
<Trigger Property="IsEnabled" Value="False"> |
||||
|
<Setter Property="Foreground" Value="{DynamicResource textHamburgerForegroundDisabled}"/> |
||||
|
</Trigger> |
||||
|
</Style.Triggers> |
||||
|
</Style> |
||||
|
</UserControl.Resources> |
||||
|
<Grid Tag="{Binding RelativeSource={RelativeSource AncestorType={x:Type UserControl}}, Path=Tag}"> |
||||
|
<StackPanel Name="hamburgerButton" |
||||
|
Width="{DynamicResource panelHamburgerMaxWidth}" |
||||
|
Height="{DynamicResource buttonHamburgerHeight}" |
||||
|
Orientation="Horizontal"> |
||||
|
<StackPanel.Background> |
||||
|
<SolidColorBrush Color="{DynamicResource buttonHamburgerBackgroundColor }" x:Name="buttonHamburgerBackgroundColor"/> |
||||
|
</StackPanel.Background> |
||||
|
<StackPanel.Style> |
||||
|
<Style TargetType="StackPanel"> |
||||
|
<Style.Triggers> |
||||
|
<Trigger Property="IsEnabled" Value="True"> |
||||
|
<Trigger.EnterActions> |
||||
|
<BeginStoryboard Name="animationHamburgerIsEnabled" Storyboard="{DynamicResource animationHamburgerApplyButtonIsEnabled}"/> |
||||
|
</Trigger.EnterActions> |
||||
|
</Trigger> |
||||
|
<Trigger Property="IsEnabled" Value="False"> |
||||
|
<Trigger.EnterActions> |
||||
|
<PauseStoryboard BeginStoryboardName="animationHamburgerIsEnabled" /> |
||||
|
<BeginStoryboard Name="animationHamburgerIsDisabled" Storyboard="{DynamicResource animationHamburgerApplyButtonIsDisabled}"/> |
||||
|
</Trigger.EnterActions> |
||||
|
|
||||
|
</Trigger> |
||||
|
</Style.Triggers> |
||||
|
</Style> |
||||
|
</StackPanel.Style> |
||||
|
<StackPanel.Triggers> |
||||
|
<EventTrigger RoutedEvent="MouseEnter"> |
||||
|
<BeginStoryboard Storyboard="{DynamicResource animationHamburgerApplyHover}" /> |
||||
|
</EventTrigger> |
||||
|
<EventTrigger RoutedEvent="MouseLeave"> |
||||
|
<BeginStoryboard Storyboard="{DynamicResource animationHamburgerApplyButtonIsEnabled}"/> |
||||
|
</EventTrigger> |
||||
|
<EventTrigger RoutedEvent="MouseLeftButtonDown"> |
||||
|
<BeginStoryboard > |
||||
|
<Storyboard> |
||||
|
<ThicknessAnimation Storyboard.TargetName="hamburgerContainer" |
||||
|
Storyboard.TargetProperty="Margin" |
||||
|
Duration="0:0:0.5" |
||||
|
From="0 0 0 0" |
||||
|
To="0 5 0 0" |
||||
|
SpeedRatio="5" |
||||
|
AutoReverse="True"/> |
||||
|
</Storyboard> |
||||
|
</BeginStoryboard> |
||||
|
</EventTrigger> |
||||
|
</StackPanel.Triggers> |
||||
|
|
||||
|
<StackPanel Name="hamburgerContainer" Orientation="Horizontal"> |
||||
|
<TextBlock Name="textIcon" FontFamily="Segoe MDL2 Assets" FontSize="{DynamicResource buttonHamburgerIconsSize}" |
||||
|
Margin="10 0 10 0" |
||||
|
VerticalAlignment="Center" |
||||
|
Text="{Binding RelativeSource={RelativeSource AncestorType={x:Type UserControl}}, Path=Icon}"/> |
||||
|
|
||||
|
<TextBlock Name="textCategory" FontSize="{DynamicResource textHamburgerSize}" |
||||
|
Margin="{Binding RelativeSource={RelativeSource AncestorType={x:Type UserControl}}, Path=TextMargin}" |
||||
|
VerticalAlignment="Center" |
||||
|
Text="{Binding RelativeSource={RelativeSource AncestorType={x:Type UserControl}}, Path=Text}"/> |
||||
|
</StackPanel> |
||||
|
</StackPanel> |
||||
|
</Grid> |
||||
|
</UserControl> |
@ -0,0 +1,61 @@ |
|||||
|
using System; |
||||
|
using System.Collections.Generic; |
||||
|
using System.Linq; |
||||
|
using System.Text; |
||||
|
using System.Threading.Tasks; |
||||
|
using System.Windows; |
||||
|
using System.Windows.Controls; |
||||
|
using System.Windows.Data; |
||||
|
using System.Windows.Documents; |
||||
|
using System.Windows.Input; |
||||
|
using System.Windows.Media; |
||||
|
using System.Windows.Media.Imaging; |
||||
|
using System.Windows.Navigation; |
||||
|
using System.Windows.Shapes; |
||||
|
|
||||
|
namespace W10SS_GUI.Controls |
||||
|
{ |
||||
|
/// <summary>
|
||||
|
/// Логика взаимодействия для HamburgerCategoryFlashButton.xaml
|
||||
|
/// </summary>
|
||||
|
public partial class HamburgerCategoryFlashButton : UserControl |
||||
|
{ |
||||
|
public HamburgerCategoryFlashButton() |
||||
|
{ |
||||
|
InitializeComponent(); |
||||
|
} |
||||
|
|
||||
|
public string Text |
||||
|
{ |
||||
|
get { return (string)GetValue(TextProperty); } |
||||
|
set { SetValue(TextProperty, value); } |
||||
|
} |
||||
|
|
||||
|
// Using a DependencyProperty as the backing store for Text. This enables animation, styling, binding, etc...
|
||||
|
public static readonly DependencyProperty TextProperty = |
||||
|
DependencyProperty.Register("Text", typeof(string), typeof(HamburgerCategoryFlashButton), new PropertyMetadata(default(string))); |
||||
|
|
||||
|
|
||||
|
public string Icon |
||||
|
{ |
||||
|
get { return (string)GetValue(IconProperty); } |
||||
|
set { SetValue(IconProperty, value); } |
||||
|
} |
||||
|
|
||||
|
// Using a DependencyProperty as the backing store for IconText. This enables animation, styling, binding, etc...
|
||||
|
public static readonly DependencyProperty IconProperty = |
||||
|
DependencyProperty.Register("Icon", typeof(string), typeof(HamburgerCategoryFlashButton), new PropertyMetadata(default(string))); |
||||
|
|
||||
|
|
||||
|
|
||||
|
public Thickness TextMargin |
||||
|
{ |
||||
|
get { return (Thickness)GetValue(TextMarginProperty); } |
||||
|
set { SetValue(TextMarginProperty, value); } |
||||
|
} |
||||
|
|
||||
|
// Using a DependencyProperty as the backing store for TextMargin. This enables animation, styling, binding, etc...
|
||||
|
public static readonly DependencyProperty TextMarginProperty = |
||||
|
DependencyProperty.Register("TextMargin", typeof(Thickness), typeof(HamburgerCategoryFlashButton), new PropertyMetadata(default(Thickness))); |
||||
|
} |
||||
|
} |
@ -0,0 +1,15 @@ |
|||||
|
<ResourceDictionary xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" |
||||
|
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" |
||||
|
xmlns:system="clr-namespace:System;assembly=mscorlib" |
||||
|
xmlns:local="clr-namespace:W10SS_GUI.Resource"> |
||||
|
<system:String x:Key="tagCategoryPrivacy">categoryPrivacy</system:String> |
||||
|
<system:String x:Key="tagCategoryUi">categoryUi</system:String> |
||||
|
<system:String x:Key="tagCategoryOneDrive">categoryOneDrive</system:String> |
||||
|
<system:String x:Key="tagCategorySystem">categorySystem</system:String> |
||||
|
<system:String x:Key="tagCategoryStartMenu">categoryStartMenu</system:String> |
||||
|
<system:String x:Key="tagCategoryUwp">categoryUwp</system:String> |
||||
|
<system:String x:Key="tagCategoryWinGame">categoryWinGame</system:String> |
||||
|
<system:String x:Key="tagCategoryTaskScheduler">categoryTaskScheduler</system:String> |
||||
|
<system:String x:Key="tagCategoryDefender">categoryDefender</system:String> |
||||
|
<system:String x:Key="tagCategoryContextMenu">categoryContextMenu</system:String> |
||||
|
</ResourceDictionary> |
Loading…
Reference in new issue