Browse Source
With this source: * A * B C D * E * F G * H I The parser was getting confused about where each unordered list actually ended when nesting the processed inner list inside the outer list. Address this by: 1) Giving "```" style code blocks their own hash just in case to make sure they can never collide with html blocks. 2) Temporarily (the indentation gets removed before final output) indent nested list tags by the current list nesting level to ensure there's no confusion about where each list/sublist starts and ends. 3) Make sure the list closing tag is followed by two newlines rather than one to avoid potentially not applying markup to the immediately following line. Since a few patterns had minor adjustments for these changes, those patterns also had a few unnecessarily capturing groups changed to non-capturing groups in the hope that some miniscule performance gain can be squeezed out with the change. Signed-off-by: Kyle J. McKay <mackyle@gmail.com>master
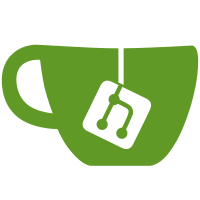
1 changed files with 25 additions and 14 deletions
Loading…
Reference in new issue