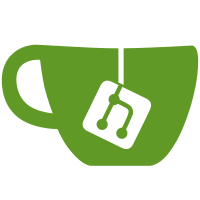
13 changed files with 269 additions and 175 deletions
@ -0,0 +1,151 @@ |
|||
'use strict'; |
|||
|
|||
|
|||
var parseLinkDestination = require('../helpers/parse_link_destination'); |
|||
var parseLinkTitle = require('../helpers/parse_link_title'); |
|||
var normalizeReference = require('../helpers/normalize_reference'); |
|||
|
|||
|
|||
module.exports = function reference(state, startLine, _endLine, silent) { |
|||
var ch, |
|||
destEndPos, |
|||
destEndLineNo, |
|||
endLine, |
|||
href, |
|||
i, |
|||
l, |
|||
label, |
|||
labelEnd, |
|||
res, |
|||
start, |
|||
str, |
|||
terminate, |
|||
terminatorRules, |
|||
title, |
|||
lines = 0, |
|||
pos = state.bMarks[startLine] + state.tShift[startLine], |
|||
max = state.eMarks[startLine], |
|||
nextLine = startLine + 1; |
|||
|
|||
if (pos >= max) { return false; } |
|||
if (state.src.charCodeAt(pos) !== 0x5B/* [ */) { return false; } |
|||
|
|||
endLine = state.lineMax; |
|||
|
|||
// jump line-by-line until empty one or EOF
|
|||
if (nextLine < endLine && !state.isEmpty(nextLine)) { |
|||
terminatorRules = state.md.block.ruler.getRules('references'); |
|||
|
|||
for (; nextLine < endLine && !state.isEmpty(nextLine); nextLine++) { |
|||
// this would be a code block normally, but after paragraph
|
|||
// it's considered a lazy continuation regardless of what's there
|
|||
if (state.tShift[nextLine] - state.blkIndent > 3) { continue; } |
|||
|
|||
// Some tags can terminate paragraph without empty line.
|
|||
terminate = false; |
|||
for (i = 0, l = terminatorRules.length; i < l; i++) { |
|||
if (terminatorRules[i](state, nextLine, endLine, true)) { |
|||
terminate = true; |
|||
break; |
|||
} |
|||
} |
|||
if (terminate) { break; } |
|||
} |
|||
} |
|||
|
|||
str = state.getLines(startLine, nextLine, state.blkIndent, false).trim(); |
|||
max = str.length; |
|||
|
|||
for (pos = 1; pos < max; pos++) { |
|||
ch = str.charCodeAt(pos); |
|||
if (ch === 0x5B /* [ */) { |
|||
return false; |
|||
} else if (ch === 0x5D /* ] */) { |
|||
labelEnd = pos; |
|||
break; |
|||
} else if (ch === 0x0A /* \n */) { |
|||
lines++; |
|||
} else if (ch === 0x5C /* \ */) { |
|||
pos++; |
|||
if (pos < max && str.charCodeAt(pos) === 0x0A) { |
|||
lines++; |
|||
} |
|||
} |
|||
} |
|||
|
|||
if (labelEnd < 0 || str.charCodeAt(labelEnd + 1) !== 0x3A/* : */) { return false; } |
|||
|
|||
// [label]: destination 'title'
|
|||
// ^^^ skip optional whitespace here
|
|||
for (pos = labelEnd + 2; pos < max; pos++) { |
|||
ch = str.charCodeAt(pos); |
|||
if (ch === 0x0A) { |
|||
lines++; |
|||
} else if (ch === 0x20) { |
|||
/*eslint no-empty:0*/ |
|||
} else { |
|||
break; |
|||
} |
|||
} |
|||
|
|||
// [label]: destination 'title'
|
|||
// ^^^^^^^^^^^ parse this
|
|||
res = parseLinkDestination(str, pos, max); |
|||
if (!res.ok) { return false; } |
|||
if (!state.md.inline.validateLink(res.str)) { return false; } |
|||
href = res.str; |
|||
pos = res.pos; |
|||
lines += res.lines; |
|||
|
|||
// save cursor state, we could require to rollback later
|
|||
destEndPos = pos; |
|||
destEndLineNo = lines; |
|||
|
|||
// [label]: destination 'title'
|
|||
// ^^^ skipping those spaces
|
|||
start = pos; |
|||
for (; pos < max; pos++) { |
|||
ch = str.charCodeAt(pos); |
|||
if (ch === 0x0A) { |
|||
lines++; |
|||
} else if (ch === 0x20) { |
|||
/*eslint no-empty:0*/ |
|||
} else { |
|||
break; |
|||
} |
|||
} |
|||
|
|||
// [label]: destination 'title'
|
|||
// ^^^^^^^ parse this
|
|||
res = parseLinkTitle(str, pos, max); |
|||
if (pos < max && start !== pos && res.ok) { |
|||
title = res.str; |
|||
pos = res.pos; |
|||
lines += res.lines; |
|||
} else { |
|||
title = ''; |
|||
pos = destEndPos; |
|||
lines = destEndLineNo; |
|||
} |
|||
|
|||
// skip trailing spaces until the rest of the line
|
|||
while (pos < max && str.charCodeAt(pos) === 0x20/* space */) { pos++; } |
|||
|
|||
if (pos < max && str.charCodeAt(pos) !== 0x0A) { |
|||
// garbage at the end of the line
|
|||
return false; |
|||
} |
|||
|
|||
if (silent) { return true; } |
|||
|
|||
label = normalizeReference(str.slice(1, labelEnd)); |
|||
if (typeof state.env.references === 'undefined') { |
|||
state.env.references = {}; |
|||
} |
|||
if (typeof state.env.references[label] === 'undefined') { |
|||
state.env.references[label] = { title: title, href: href }; |
|||
} |
|||
|
|||
state.line = startLine + lines + 1; |
|||
return true; |
|||
}; |
@ -1,107 +0,0 @@ |
|||
'use strict'; |
|||
|
|||
|
|||
var parseLinkDestination = require('../helpers/parse_link_destination'); |
|||
var parseLinkTitle = require('../helpers/parse_link_title'); |
|||
var normalizeReference = require('../helpers/normalize_reference'); |
|||
|
|||
|
|||
function parseReference(str, md, env) { |
|||
var state, pos, code, start, href, title, label, ch, max, |
|||
labelEnd = -1; |
|||
|
|||
if (str.charCodeAt(0) !== 0x5B/* [ */) { return -1; } |
|||
|
|||
if (str.indexOf(']:') === -1) { return -1; } |
|||
|
|||
state = new md.inline.State(str, md, env, []); |
|||
max = state.posMax; |
|||
|
|||
for (pos = 1; pos < max; pos++) { |
|||
ch = str.charCodeAt(pos); |
|||
if (ch === 0x5B /* [ */) { |
|||
return -1; |
|||
} else if (ch === 0x5D /* ] */) { |
|||
labelEnd = pos; |
|||
break; |
|||
} else if (ch === 0x5C /* \ */) { |
|||
pos++; |
|||
} |
|||
} |
|||
|
|||
if (labelEnd < 0 || str.charCodeAt(labelEnd + 1) !== 0x3A/* : */) { return -1; } |
|||
|
|||
// [label]: destination 'title'
|
|||
// ^^^ skip optional whitespace here
|
|||
for (pos = labelEnd + 2; pos < max; pos++) { |
|||
code = state.src.charCodeAt(pos); |
|||
if (code !== 0x20 && code !== 0x0A) { break; } |
|||
} |
|||
|
|||
// [label]: destination 'title'
|
|||
// ^^^^^^^^^^^ parse this
|
|||
if (!parseLinkDestination(state, pos)) { return -1; } |
|||
href = state.linkContent; |
|||
pos = state.pos; |
|||
|
|||
// [label]: destination 'title'
|
|||
// ^^^ skipping those spaces
|
|||
start = pos; |
|||
for (pos = pos + 1; pos < max; pos++) { |
|||
code = state.src.charCodeAt(pos); |
|||
if (code !== 0x20 && code !== 0x0A) { break; } |
|||
} |
|||
|
|||
// [label]: destination 'title'
|
|||
// ^^^^^^^ parse this
|
|||
if (pos < max && start !== pos && parseLinkTitle(state, pos)) { |
|||
title = state.linkContent; |
|||
pos = state.pos; |
|||
} else { |
|||
title = ''; |
|||
pos = start; |
|||
} |
|||
|
|||
// ensure that the end of the line is empty
|
|||
while (pos < max && state.src.charCodeAt(pos) === 0x20/* space */) { pos++; } |
|||
if (pos < max && state.src.charCodeAt(pos) !== 0x0A) { return -1; } |
|||
|
|||
label = normalizeReference(str.slice(1, labelEnd)); |
|||
if (typeof env.references[label] === 'undefined') { |
|||
env.references[label] = { title: title, href: href }; |
|||
} |
|||
|
|||
return pos; |
|||
} |
|||
|
|||
|
|||
module.exports = function references(state) { |
|||
var tokens = state.tokens, i, l, content, pos; |
|||
|
|||
state.env.references = state.env.references || {}; |
|||
|
|||
if (state.inlineMode) { |
|||
return; |
|||
} |
|||
|
|||
// Scan definitions in paragraph inlines
|
|||
for (i = 1, l = tokens.length - 1; i < l; i++) { |
|||
if (tokens[i].type === 'inline' && |
|||
tokens[i - 1].type === 'paragraph_open' && |
|||
tokens[i + 1].type === 'paragraph_close') { |
|||
|
|||
content = tokens[i].content; |
|||
while (content.length) { |
|||
pos = parseReference(content, state.md, state.env); |
|||
if (pos < 0) { break; } |
|||
content = content.slice(pos).trim(); |
|||
} |
|||
|
|||
tokens[i].content = content; |
|||
if (!content.length) { |
|||
tokens[i - 1].tight = true; |
|||
tokens[i + 1].tight = true; |
|||
} |
|||
} |
|||
} |
|||
}; |
Loading…
Reference in new issue