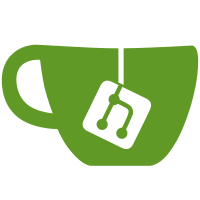
10 changed files with 6 additions and 613 deletions
@ -1,101 +0,0 @@ |
|||
'use strict'; |
|||
|
|||
|
|||
// parse sequence of markers,
|
|||
// "start" should point at a valid marker
|
|||
function scanDelims(state, start) { |
|||
var pos = start, lastChar, nextChar, count, |
|||
can_open = true, |
|||
can_close = true, |
|||
max = state.posMax, |
|||
marker = state.src.charCodeAt(start); |
|||
|
|||
lastChar = start > 0 ? state.src.charCodeAt(start - 1) : -1; |
|||
|
|||
while (pos < max && state.src.charCodeAt(pos) === marker) { pos++; } |
|||
if (pos >= max) { can_open = false; } |
|||
count = pos - start; |
|||
|
|||
nextChar = pos < max ? state.src.charCodeAt(pos) : -1; |
|||
|
|||
// check whitespace conditions
|
|||
if (nextChar === 0x20 || nextChar === 0x0A) { can_open = false; } |
|||
if (lastChar === 0x20 || lastChar === 0x0A) { can_close = false; } |
|||
|
|||
return { |
|||
can_open: can_open, |
|||
can_close: can_close, |
|||
delims: count |
|||
}; |
|||
} |
|||
|
|||
module.exports = function(state, silent) { |
|||
var startCount, |
|||
count, |
|||
tagCount, |
|||
found, |
|||
stack, |
|||
res, |
|||
max = state.posMax, |
|||
start = state.pos, |
|||
marker = state.src.charCodeAt(start); |
|||
|
|||
if (marker !== 0x2B/* + */) { return false; } |
|||
if (silent) { return false; } // don't run any pairs in validation mode
|
|||
|
|||
res = scanDelims(state, start); |
|||
startCount = res.delims; |
|||
if (!res.can_open) { |
|||
state.pos += startCount; |
|||
if (!silent) { state.pending += state.src.slice(start, state.pos); } |
|||
return true; |
|||
} |
|||
|
|||
stack = Math.floor(startCount / 2); |
|||
if (stack <= 0) { return false; } |
|||
state.pos = start + startCount; |
|||
|
|||
while (state.pos < max) { |
|||
if (state.src.charCodeAt(state.pos) === marker) { |
|||
res = scanDelims(state, state.pos); |
|||
count = res.delims; |
|||
tagCount = Math.floor(count / 2); |
|||
if (res.can_close) { |
|||
if (tagCount >= stack) { |
|||
state.pos += count - 2; |
|||
found = true; |
|||
break; |
|||
} |
|||
stack -= tagCount; |
|||
state.pos += count; |
|||
continue; |
|||
} |
|||
|
|||
if (res.can_open) { stack += tagCount; } |
|||
state.pos += count; |
|||
continue; |
|||
} |
|||
|
|||
state.md.inline.skipToken(state); |
|||
} |
|||
|
|||
if (!found) { |
|||
// parser failed to find ending tag, so it's not valid emphasis
|
|||
state.pos = start; |
|||
return false; |
|||
} |
|||
|
|||
// found!
|
|||
state.posMax = state.pos; |
|||
state.pos = start + 2; |
|||
|
|||
if (!silent) { |
|||
state.push({ type: 'ins_open', level: state.level++ }); |
|||
state.md.inline.tokenize(state); |
|||
state.push({ type: 'ins_close', level: --state.level }); |
|||
} |
|||
|
|||
state.pos = state.posMax + 2; |
|||
state.posMax = max; |
|||
return true; |
|||
}; |
@ -1,101 +0,0 @@ |
|||
'use strict'; |
|||
|
|||
|
|||
// parse sequence of markers,
|
|||
// "start" should point at a valid marker
|
|||
function scanDelims(state, start) { |
|||
var pos = start, lastChar, nextChar, count, |
|||
can_open = true, |
|||
can_close = true, |
|||
max = state.posMax, |
|||
marker = state.src.charCodeAt(start); |
|||
|
|||
lastChar = start > 0 ? state.src.charCodeAt(start - 1) : -1; |
|||
|
|||
while (pos < max && state.src.charCodeAt(pos) === marker) { pos++; } |
|||
if (pos >= max) { can_open = false; } |
|||
count = pos - start; |
|||
|
|||
nextChar = pos < max ? state.src.charCodeAt(pos) : -1; |
|||
|
|||
// check whitespace conditions
|
|||
if (nextChar === 0x20 || nextChar === 0x0A) { can_open = false; } |
|||
if (lastChar === 0x20 || lastChar === 0x0A) { can_close = false; } |
|||
|
|||
return { |
|||
can_open: can_open, |
|||
can_close: can_close, |
|||
delims: count |
|||
}; |
|||
} |
|||
|
|||
module.exports = function(state, silent) { |
|||
var startCount, |
|||
count, |
|||
tagCount, |
|||
found, |
|||
stack, |
|||
res, |
|||
max = state.posMax, |
|||
start = state.pos, |
|||
marker = state.src.charCodeAt(start); |
|||
|
|||
if (marker !== 0x3D/* = */) { return false; } |
|||
if (silent) { return false; } // don't run any pairs in validation mode
|
|||
|
|||
res = scanDelims(state, start); |
|||
startCount = res.delims; |
|||
if (!res.can_open) { |
|||
state.pos += startCount; |
|||
if (!silent) { state.pending += state.src.slice(start, state.pos); } |
|||
return true; |
|||
} |
|||
|
|||
stack = Math.floor(startCount / 2); |
|||
if (stack <= 0) { return false; } |
|||
state.pos = start + startCount; |
|||
|
|||
while (state.pos < max) { |
|||
if (state.src.charCodeAt(state.pos) === marker) { |
|||
res = scanDelims(state, state.pos); |
|||
count = res.delims; |
|||
tagCount = Math.floor(count / 2); |
|||
if (res.can_close) { |
|||
if (tagCount >= stack) { |
|||
state.pos += count - 2; |
|||
found = true; |
|||
break; |
|||
} |
|||
stack -= tagCount; |
|||
state.pos += count; |
|||
continue; |
|||
} |
|||
|
|||
if (res.can_open) { stack += tagCount; } |
|||
state.pos += count; |
|||
continue; |
|||
} |
|||
|
|||
state.md.inline.skipToken(state); |
|||
} |
|||
|
|||
if (!found) { |
|||
// parser failed to find ending tag, so it's not valid emphasis
|
|||
state.pos = start; |
|||
return false; |
|||
} |
|||
|
|||
// found!
|
|||
state.posMax = state.pos; |
|||
state.pos = start + 2; |
|||
|
|||
if (!silent) { |
|||
state.push({ type: 'mark_open', level: state.level++ }); |
|||
state.md.inline.tokenize(state); |
|||
state.push({ type: 'mark_close', level: --state.level }); |
|||
} |
|||
|
|||
state.pos = state.posMax + 2; |
|||
state.posMax = max; |
|||
return true; |
|||
}; |
@ -1,59 +0,0 @@ |
|||
// Process ~subscript~
|
|||
|
|||
'use strict'; |
|||
|
|||
// same as UNESCAPE_MD_RE plus a space
|
|||
var UNESCAPE_RE = /\\([ \\!"#$%&'()*+,.\/:;<=>?@[\]^_`{|}~-])/g; |
|||
|
|||
module.exports = function sub(state, silent) { |
|||
var found, |
|||
content, |
|||
max = state.posMax, |
|||
start = state.pos; |
|||
|
|||
if (state.src.charCodeAt(start) !== 0x7E/* ~ */) { return false; } |
|||
if (silent) { return false; } // don't run any pairs in validation mode
|
|||
if (start + 2 >= max) { return false; } |
|||
|
|||
state.pos = start + 1; |
|||
|
|||
while (state.pos < max) { |
|||
if (state.src.charCodeAt(state.pos) === 0x7E/* ~ */) { |
|||
found = true; |
|||
break; |
|||
} |
|||
|
|||
state.md.inline.skipToken(state); |
|||
} |
|||
|
|||
if (!found || start + 1 === state.pos) { |
|||
state.pos = start; |
|||
return false; |
|||
} |
|||
|
|||
content = state.src.slice(start + 1, state.pos); |
|||
|
|||
// don't allow unescaped spaces/newlines inside
|
|||
if (content.match(/(^|[^\\])(\\\\)*\s/)) { |
|||
state.pos = start; |
|||
return false; |
|||
} |
|||
|
|||
// found!
|
|||
state.posMax = state.pos; |
|||
state.pos = start + 1; |
|||
|
|||
if (!silent) { |
|||
state.push({ type: 'sub_open', level: state.level++ }); |
|||
state.push({ |
|||
type: 'text', |
|||
level: state.level, |
|||
content: content.replace(UNESCAPE_RE, '$1') |
|||
}); |
|||
state.push({ type: 'sub_close', level: --state.level }); |
|||
} |
|||
|
|||
state.pos = state.posMax + 1; |
|||
state.posMax = max; |
|||
return true; |
|||
}; |
@ -1,59 +0,0 @@ |
|||
// Process ^superscript^
|
|||
|
|||
'use strict'; |
|||
|
|||
// same as UNESCAPE_MD_RE plus a space
|
|||
var UNESCAPE_RE = /\\([ \\!"#$%&'()*+,.\/:;<=>?@[\]^_`{|}~-])/g; |
|||
|
|||
module.exports = function sup(state, silent) { |
|||
var found, |
|||
content, |
|||
max = state.posMax, |
|||
start = state.pos; |
|||
|
|||
if (state.src.charCodeAt(start) !== 0x5E/* ^ */) { return false; } |
|||
if (silent) { return false; } // don't run any pairs in validation mode
|
|||
if (start + 2 >= max) { return false; } |
|||
|
|||
state.pos = start + 1; |
|||
|
|||
while (state.pos < max) { |
|||
if (state.src.charCodeAt(state.pos) === 0x5E/* ^ */) { |
|||
found = true; |
|||
break; |
|||
} |
|||
|
|||
state.md.inline.skipToken(state); |
|||
} |
|||
|
|||
if (!found || start + 1 === state.pos) { |
|||
state.pos = start; |
|||
return false; |
|||
} |
|||
|
|||
content = state.src.slice(start + 1, state.pos); |
|||
|
|||
// don't allow unescaped spaces/newlines inside
|
|||
if (content.match(/(^|[^\\])(\\\\)*\s/)) { |
|||
state.pos = start; |
|||
return false; |
|||
} |
|||
|
|||
// found!
|
|||
state.posMax = state.pos; |
|||
state.pos = start + 1; |
|||
|
|||
if (!silent) { |
|||
state.push({ type: 'sup_open', level: state.level++ }); |
|||
state.push({ |
|||
type: 'text', |
|||
level: state.level, |
|||
content: content.replace(UNESCAPE_RE, '$1') |
|||
}); |
|||
state.push({ type: 'sup_close', level: --state.level }); |
|||
} |
|||
|
|||
state.pos = state.posMax + 1; |
|||
state.posMax = max; |
|||
return true; |
|||
}; |
@ -1,104 +0,0 @@ |
|||
. |
|||
++Insert++ |
|||
. |
|||
<p><ins>Insert</ins></p> |
|||
. |
|||
|
|||
|
|||
. |
|||
x ++++foo++ bar++ |
|||
. |
|||
<p>x <ins><ins>foo</ins> bar</ins></p> |
|||
. |
|||
|
|||
. |
|||
x ++foo ++bar++++ |
|||
. |
|||
<p>x <ins>foo <ins>bar</ins></ins></p> |
|||
. |
|||
|
|||
. |
|||
x ++++foo++++ |
|||
. |
|||
<p>x <ins><ins>foo</ins></ins></p> |
|||
. |
|||
|
|||
. |
|||
x +++foo+++ |
|||
. |
|||
<p>x <ins>+foo+</ins></p> |
|||
. |
|||
|
|||
Inserts have the same priority as emphases: |
|||
|
|||
. |
|||
**++test**++ |
|||
|
|||
++**test++** |
|||
. |
|||
<p><strong>++test</strong>++</p> |
|||
<p><ins>**test</ins>**</p> |
|||
. |
|||
|
|||
Inserts have the same priority as emphases with respect to links: |
|||
. |
|||
[++link]()++ |
|||
|
|||
++[link++]() |
|||
. |
|||
<p><a href="">++link</a>++</p> |
|||
<p>++<a href="">link++</a></p> |
|||
. |
|||
|
|||
Inserts have the same priority as emphases with respect to backticks: |
|||
. |
|||
++`code++` |
|||
|
|||
`++code`++ |
|||
. |
|||
<p>++<code>code++</code></p> |
|||
<p><code>++code</code>++</p> |
|||
. |
|||
|
|||
Nested inserts: |
|||
. |
|||
++foo ++bar++ baz++ |
|||
. |
|||
<p><ins>foo <ins>bar</ins> baz</ins></p> |
|||
. |
|||
|
|||
. |
|||
++f **o ++o b++ a** r++ |
|||
. |
|||
<p><ins>f <strong>o <ins>o b</ins> a</strong> r</ins></p> |
|||
. |
|||
|
|||
Should not have a whitespace between text and "++": |
|||
. |
|||
foo ++ bar ++ baz |
|||
. |
|||
<p>foo ++ bar ++ baz</p> |
|||
. |
|||
|
|||
|
|||
Newline should be considered a whitespace: |
|||
|
|||
. |
|||
++test |
|||
++ |
|||
|
|||
++ |
|||
test++ |
|||
|
|||
++ |
|||
test |
|||
++ |
|||
. |
|||
<p>++test |
|||
++</p> |
|||
<p>++ |
|||
test++</p> |
|||
<p>++ |
|||
test |
|||
++</p> |
|||
. |
@ -1,102 +0,0 @@ |
|||
. |
|||
==Mark== |
|||
. |
|||
<p><mark>Mark</mark></p> |
|||
. |
|||
|
|||
. |
|||
x ====foo== bar== |
|||
. |
|||
<p>x <mark><mark>foo</mark> bar</mark></p> |
|||
. |
|||
|
|||
. |
|||
x ==foo ==bar==== |
|||
. |
|||
<p>x <mark>foo <mark>bar</mark></mark></p> |
|||
. |
|||
|
|||
. |
|||
x ====foo==== |
|||
. |
|||
<p>x <mark><mark>foo</mark></mark></p> |
|||
. |
|||
|
|||
. |
|||
x ===foo=== |
|||
. |
|||
<p>x <mark>=foo=</mark></p> |
|||
. |
|||
|
|||
Marks have the same priority as emphases: |
|||
|
|||
. |
|||
**==test**== |
|||
|
|||
==**test==** |
|||
. |
|||
<p><strong>==test</strong>==</p> |
|||
<p><mark>**test</mark>**</p> |
|||
. |
|||
|
|||
Marks have the same priority as emphases with respect to links: |
|||
. |
|||
[==link]()== |
|||
|
|||
==[link==]() |
|||
. |
|||
<p><a href="">==link</a>==</p> |
|||
<p>==<a href="">link==</a></p> |
|||
. |
|||
|
|||
Marks have the same priority as emphases with respect to backticks: |
|||
. |
|||
==`code==` |
|||
|
|||
`==code`== |
|||
. |
|||
<p>==<code>code==</code></p> |
|||
<p><code>==code</code>==</p> |
|||
. |
|||
|
|||
Nested marks: |
|||
. |
|||
==foo ==bar== baz== |
|||
. |
|||
<p><mark>foo <mark>bar</mark> baz</mark></p> |
|||
. |
|||
|
|||
. |
|||
==f **o ==o b== a** r== |
|||
. |
|||
<p><mark>f <strong>o <mark>o b</mark> a</strong> r</mark></p> |
|||
. |
|||
|
|||
Should not have a whitespace between text and "==": |
|||
. |
|||
foo == bar == baz |
|||
. |
|||
<p>foo == bar == baz</p> |
|||
. |
|||
|
|||
|
|||
Newline should be considered a whitespace: |
|||
|
|||
. |
|||
==test |
|||
== |
|||
|
|||
== |
|||
test== |
|||
|
|||
== |
|||
test |
|||
== |
|||
. |
|||
<h1>==test</h1> |
|||
<p>== |
|||
test==</p> |
|||
<p>== |
|||
test |
|||
==</p> |
|||
. |
@ -1,36 +0,0 @@ |
|||
|
|||
. |
|||
~foo\~ |
|||
. |
|||
<p>~foo~</p> |
|||
. |
|||
|
|||
. |
|||
~foo bar~ |
|||
. |
|||
<p>~foo bar~</p> |
|||
. |
|||
|
|||
. |
|||
~foo\ bar\ baz~ |
|||
. |
|||
<p><sub>foo bar baz</sub></p> |
|||
. |
|||
|
|||
. |
|||
~\ foo\ ~ |
|||
. |
|||
<p><sub> foo </sub></p> |
|||
. |
|||
|
|||
. |
|||
~foo\\\\\\\ bar~ |
|||
. |
|||
<p><sub>foo\\\ bar</sub></p> |
|||
. |
|||
|
|||
. |
|||
~foo\\\\\\ bar~ |
|||
. |
|||
<p>~foo\\\ bar~</p> |
|||
. |
@ -1,42 +0,0 @@ |
|||
|
|||
. |
|||
^test^ |
|||
. |
|||
<p><sup>test</sup></p> |
|||
. |
|||
|
|||
. |
|||
^foo\^ |
|||
. |
|||
<p>^foo^</p> |
|||
. |
|||
|
|||
. |
|||
2^4 + 3^5 |
|||
. |
|||
<p>2^4 + 3^5</p> |
|||
. |
|||
|
|||
. |
|||
^foo~bar^baz^bar~foo^ |
|||
. |
|||
<p><sup>foo~bar</sup>baz<sup>bar~foo</sup></p> |
|||
. |
|||
|
|||
. |
|||
^\ foo\ ^ |
|||
. |
|||
<p><sup> foo </sup></p> |
|||
. |
|||
|
|||
. |
|||
^foo\\\\\\\ bar^ |
|||
. |
|||
<p><sup>foo\\\ bar</sup></p> |
|||
. |
|||
|
|||
. |
|||
^foo\\\\\\ bar^ |
|||
. |
|||
<p>^foo\\\ bar^</p> |
|||
. |
Loading…
Reference in new issue