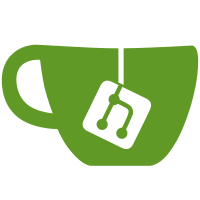
4 changed files with 174 additions and 47 deletions
@ -1,67 +1,199 @@ |
|||
<!-- styles hack until ndoc updated --> |
|||
<style> |
|||
header .name_prefix { font-weight: normal; } |
|||
</style> |
|||
# markdown-it |
|||
|
|||
# markdown-it API |
|||
## Install |
|||
|
|||
### Simple use |
|||
**node.js** & **bower**: |
|||
|
|||
```bash |
|||
npm install markdown-it --save |
|||
bower install markdown-it --save |
|||
``` |
|||
|
|||
**browser (CDN):** |
|||
|
|||
- [jsDeliver CDN](http://www.jsdelivr.com/#!markdown-it "jsDelivr CDN") |
|||
- [cdnjs.com CDN](https://cdnjs.com/libraries/markdown-it "cdnjs.com") |
|||
|
|||
|
|||
## Usage examples |
|||
|
|||
See also: |
|||
|
|||
- [Development info](https://github.com/markdown-it/markdown-it/tree/master/docs) - |
|||
for plugins writers. |
|||
|
|||
In most cases you will use `markdown-it` in very simple way: |
|||
|
|||
```javascript |
|||
### Simple |
|||
|
|||
```js |
|||
// node.js, "classic" way: |
|||
var MarkdownIt = require('markdown-it'), |
|||
md = new MarkdownIt(); |
|||
var result = md.render('# markdown-it rulezz!'); |
|||
|
|||
// node.js, the same, but with sugar: |
|||
var md = require('markdown-it')(); |
|||
var result = md.render('# markdown-it rulezz!'); |
|||
|
|||
// browser without AMD, added to "window" on script load |
|||
// Note, there is no dash in "markdownit". |
|||
var md = window.markdownit(); |
|||
var result = md.render('# markdown-it rulezz!'); |
|||
``` |
|||
|
|||
var result = md.render('your_markdown_string'); |
|||
Single line rendering, without paragraph wrap: |
|||
|
|||
// Or for inline (without paragraths & blocks) |
|||
var resultInline = md.renderInline('your_markdown_inline_string'); |
|||
```js |
|||
var md = require('markdown-it')(); |
|||
var result = md.renderInline('__markdown-it__ rulezz!'); |
|||
``` |
|||
|
|||
### Advanced use |
|||
|
|||
Advanced use consist of this steps: |
|||
### Init with presets and options |
|||
|
|||
1. Create instance with desired preset & options. |
|||
2. Add plugins. |
|||
3. Enable/Disable additional rules. |
|||
4. Rewrite renderer functions. |
|||
5. Use result to call `.render()` or `.renderInline()` method. |
|||
(*) presets define combinations of active rules and options. Can be |
|||
`"commonmark"`, `"zero"` or `"default"` (if skipped). See |
|||
[API docs](https://markdown-it.github.io/markdown-it/#MarkdownIt.new) for more details. |
|||
|
|||
Of cause, you can skip not needed steps, or change sequense. |
|||
```js |
|||
// commonmark mode |
|||
var md = require('markdown-it')('commonmark'); |
|||
|
|||
// default mode |
|||
var md = require('markdown-it')(); |
|||
|
|||
__Example 1.__ Minimalistic mode with bold, italic and line breaks: |
|||
// enable everything |
|||
var md = require('markdown-it')({ |
|||
html: true, |
|||
linkify: true, |
|||
typographer: true |
|||
}); |
|||
|
|||
// full options list (defaults) |
|||
var md = require('markdown-it')({ |
|||
html: false, // Enable HTML tags in source |
|||
xhtmlOut: false, // Use '/' to close single tags (<br />). |
|||
// This is only for full CommonMark compatibility. |
|||
breaks: false, // Convert '\n' in paragraphs into <br> |
|||
langPrefix: 'language-', // CSS language prefix for fenced blocks. Can be |
|||
// useful for external highlighters. |
|||
linkify: false, // Autoconvert URL-like text to links |
|||
|
|||
// Enable some language-neutral replacement + quotes beautification |
|||
typographer: false, |
|||
|
|||
// Double + single quotes replacement pairs, when typographer enabled, |
|||
// and smartquotes on. Could be either a String or an Array. |
|||
// |
|||
// For example, you can use '«»„“' for Russian, '„“‚‘' for German, |
|||
// and ['«\xA0', '\xA0»', '‹\xA0', '\xA0›'] for French (including nbsp). |
|||
quotes: '“”‘’', |
|||
|
|||
// Highlighter function. Should return escaped HTML, |
|||
// or '' if the source string is not changed and should be escaped externaly. |
|||
// If result starts with <pre... internal wrapper is skipped. |
|||
highlight: function (/*str, lang*/) { return ''; } |
|||
}); |
|||
``` |
|||
|
|||
```javascript |
|||
var md = require('markdown-it')('zero', { breaks: true }) |
|||
.enable([ 'newline', 'emphasis' ]); |
|||
### Plugins load |
|||
|
|||
var result = md.renderInline(...); |
|||
```js |
|||
var md = require('markdown-it')() |
|||
.use(plugin1) |
|||
.use(plugin2, opts, ...) |
|||
.use(plugin3); |
|||
``` |
|||
|
|||
|
|||
__Example 2.__ Load plugin and disable tables: |
|||
### Syntax highlighting |
|||
|
|||
```javascript |
|||
var md = require('markdown-it')() |
|||
.use(require('markdown-it-emoji')) |
|||
.disable('table'); |
|||
Apply syntax highlighting to fenced code blocks with the `highlight` option: |
|||
|
|||
var result = md.render(...); |
|||
``` |
|||
```js |
|||
var hljs = require('highlight.js') // https://highlightjs.org/ |
|||
|
|||
// Actual default values |
|||
var md = require('markdown-it')({ |
|||
highlight: function (str, lang) { |
|||
if (lang && hljs.getLanguage(lang)) { |
|||
try { |
|||
return hljs.highlight(lang, str).value; |
|||
} catch (__) {} |
|||
} |
|||
|
|||
__Example 3.__ Replace `<strong>` with `<b>` in rendered result: |
|||
return ''; // use external default escaping |
|||
} |
|||
}); |
|||
``` |
|||
|
|||
```javascript |
|||
var md = require('markdown-it')(); |
|||
Or with full wrapper override (if you need assign class to `<pre>`): |
|||
|
|||
```js |
|||
var hljs = require('highlight.js') // https://highlightjs.org/ |
|||
|
|||
// Actual default values |
|||
var md = require('markdown-it')({ |
|||
highlight: function (str, lang) { |
|||
if (lang && hljs.getLanguage(lang)) { |
|||
try { |
|||
return '<pre class="hljs"><code>' + |
|||
hljs.highlight(lang, str, true).value + |
|||
'</code></pre>'; |
|||
} catch (__) {} |
|||
} |
|||
|
|||
return '<pre class="hljs"><code>' + md.utils.escapeHtml(str) + '</code></pre>'; |
|||
} |
|||
}); |
|||
``` |
|||
|
|||
### Linkify |
|||
|
|||
md.renderer.rules.strong_open = function () { return '<b>'; }; |
|||
md.renderer.rules.strong_close = function () { return '</b>'; }; |
|||
`linkify: true` uses [linkify-it](https://github.com/markdown-it/linkify-it). To |
|||
configure linkify-it, access the linkify instance through `md.linkify`: |
|||
|
|||
var result = md.renderInline(...); |
|||
```js |
|||
md.linkify.tlds('.py', false); // disables .py as top level domain |
|||
``` |
|||
|
|||
## Syntax extensions |
|||
|
|||
Embedded (enabled by default): |
|||
|
|||
- [Tables](https://help.github.com/articles/github-flavored-markdown/#tables) (GFM) |
|||
- [Strikethrough](https://help.github.com/articles/github-flavored-markdown/#strikethrough) (GFM) |
|||
|
|||
See classes doc for all available features and more examples. |
|||
Via plugins: |
|||
|
|||
- [subscript](https://github.com/markdown-it/markdown-it-sub) |
|||
- [superscript](https://github.com/markdown-it/markdown-it-sup) |
|||
- [footnote](https://github.com/markdown-it/markdown-it-footnote) |
|||
- [definition list](https://github.com/markdown-it/markdown-it-deflist) |
|||
- [abbreviation](https://github.com/markdown-it/markdown-it-abbr) |
|||
- [emoji](https://github.com/markdown-it/markdown-it-emoji) |
|||
- [custom container](https://github.com/markdown-it/markdown-it-container) |
|||
- [insert](https://github.com/markdown-it/markdown-it-ins) |
|||
- [mark](https://github.com/markdown-it/markdown-it-mark) |
|||
- ... and [others](https://www.npmjs.org/browse/keyword/markdown-it-plugin) |
|||
|
|||
|
|||
### Manage rules |
|||
|
|||
By default all rules are enabled, but can be restricted by options. On plugin |
|||
load all its rules are enabled automatically. |
|||
|
|||
```js |
|||
// Activate/deactivate rules, with curring |
|||
var md = require('markdown-it')() |
|||
.disable([ 'link', 'image' ]) |
|||
.enable([ 'link' ]) |
|||
.enable('image'); |
|||
|
|||
// Enable everything |
|||
md = require('markdown-it')('full', { |
|||
html: true, |
|||
linkify: true, |
|||
typographer: true, |
|||
}); |
|||
``` |
|||
|
Loading…
Reference in new issue